Just a couple of ways to get the System Fonts using JavaScript. There are ref links below with demos.
Getting System Fonts Using JavaScript
try {
//adds a swf to the page and reads fonts
$('body').append('');
$('#flashcontent').flash(
{
"src": "resources/FontList.swf",
"width": "1",
"height": "1",
"swliveconnect": "true",
"id": "flashfontshelper",
"name": "flashfontshelper"
},
{ update: false });
//timeout required for swf to settle.
setTimeout(function()
{
console.log(window.fonts);
return window.fonts;
}, _this.settings.flashDelay);
}
catch(ex) {
_this.errors.push('No fonts detected.');
return fonts;
}
Source Demo
Another way
try {
//adds a swf to the page and reads fonts
$('body').append('').flash(
{
"src": "resources/fonts.swf",
"width": "1",
"height": "1",
"swliveconnect": "true",
"id": "flashfontshelper",
"name": "flashfontshelper"
},
{ update: false });
//timeout required for swf to settle.
setTimeout(function()
{
var fonts = "",
obj = document.getElementById("flashfontshelper");
//get fonts
if (obj && typeof(obj.GetVariable) != "undefined") {
fonts = obj.GetVariable("/:user_fonts").replace(/,/g,", ");
}
if (!fonts) {
fonts = "No Flash or Java fonts detected";
_this.errors.push('No Flash or Java fonts detected.');
}
console.log(fonts);
return fonts;
}, 100);
return true;
}
catch(ex) {
fonts = this.errors.push('No Flash or Java fonts detected.');
}
errors:
Uncaught TypeError: Property ‘fontList’ of object [object Object] is not a function
Demo
Frequently Asked Questions (FAQs) about System Fonts in JavaScript
How can I list all the fonts a user’s browser can display using JavaScript?
JavaScript does not have a built-in method to list all the fonts a user’s browser can display. However, you can use a JavaScript library like “FontFaceSet” to load and check if a font is available. You can also use the “document.fonts.check()” method to check if a specific font is available. Remember, due to privacy concerns, browsers do not allow scripts to directly access the list of installed fonts.
What are web-safe fonts and how can I use them in CSS?
Web-safe fonts are fonts that are likely to be present on a wide range of computer systems, and thus are ideal for use on the web. These include fonts like Arial, Times New Roman, and Courier New. In CSS, you can use these fonts by specifying them in the “font-family” property. For example, “font-family: Arial, sans-serif;”. The browser will use the first font in the list that is installed on the user’s system.
What is the Local Font Access API and how can I use it?
The Local Font Access API is a web API that allows you to access the user’s locally installed fonts. However, due to privacy concerns, this API is not widely supported and its use is generally discouraged. If you still want to use it, you can do so by calling the “navigator.fonts.query()” method, which returns a promise that resolves with a list of FontMetadata objects representing the user’s installed fonts.
How can I use HTML and CSS to specify web-safe fonts?
In HTML and CSS, you can specify web-safe fonts using the “font-family” property. You should list the fonts in order of preference, separated by commas. The browser will use the first font in the list that is installed on the user’s system. For example, “font-family: Arial, Helvetica, sans-serif;”.
How can I use a JavaScript library to detect the user’s installed fonts?
There are several JavaScript libraries available that can detect the user’s installed fonts, such as “FontFaceSet” and “FontDetector”. These libraries work by loading a font and then checking if it is available. However, due to privacy concerns, the use of such libraries is generally discouraged.
What are the most common web-safe fonts?
The most common web-safe fonts include Arial, Times New Roman, Courier New, Georgia, and Verdana. These fonts are likely to be installed on a wide range of computer systems, making them ideal for use on the web.
How can I check if a specific font is installed using JavaScript?
You can check if a specific font is installed using the “document.fonts.check()” method in JavaScript. This method returns a boolean value indicating whether the font is available. For example, “document.fonts.check(’16px Arial’)” will return true if Arial is installed.
Can I access the user’s installed fonts directly using JavaScript?
Due to privacy concerns, browsers do not allow scripts to directly access the list of installed fonts. However, you can use methods like “document.fonts.check()” to check if a specific font is available.
How can I specify a fallback font in CSS?
In CSS, you can specify a fallback font by listing multiple fonts in the “font-family” property, separated by commas. The browser will use the first font in the list that is installed on the user’s system. If none of the listed fonts are installed, the browser will use a default font.
What are the privacy concerns associated with accessing the user’s installed fonts?
Accessing the user’s installed fonts can be a privacy concern because it can be used for fingerprinting, a technique that allows websites to uniquely identify and track users based on their system configuration. For this reason, browsers do not allow scripts to directly access the list of installed fonts.
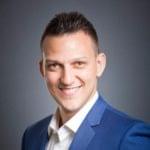
Sam Deering has 15+ years of programming and website development experience. He was a website consultant at Console, ABC News, Flight Centre, Sapient Nitro, and the QLD Government and runs a tech blog with over 1 million views per month. Currently, Sam is the Founder of Crypto News, Australia.